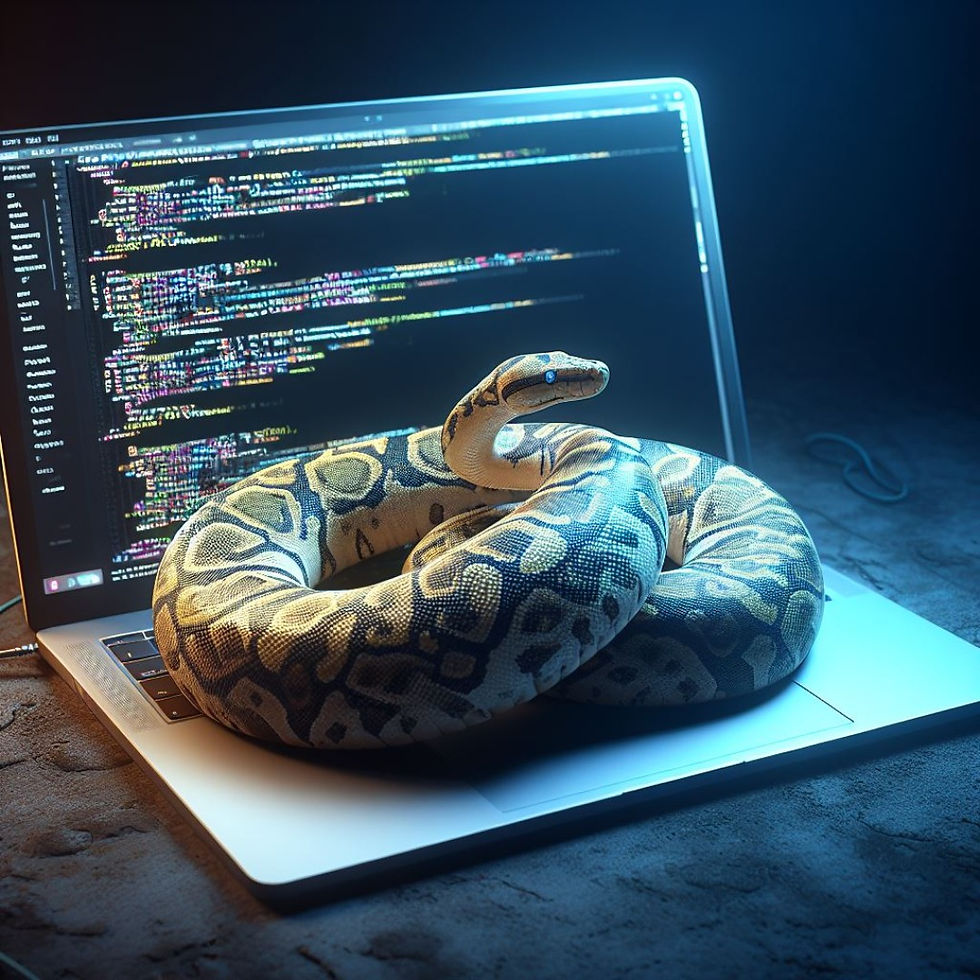
A quick and easy script.
Method 1.
import subprocess
# Define the network interface and the new MAC address
interface = "eth0" # Replace with the name of your network interface new_mac = "00:11:22:33:44:55" # Replace with the desired MAC address
# Disable the network interface
subprocess.call(["ifconfig", interface, "down"])
# Change the MAC address
subprocess.call(["ifconfig", interface, "hw", "ether", new_mac])
# Enable the network interface
subprocess.call(["ifconfig", interface, "up"])
print(f"MAC address of {interface} changed to {new_mac}")
Step 1: Open a Python code editor or create a new Python script file.
Step 2: Copy and paste the code provided above into the script.
Step 3: Replace "eth0" with the name of your network interface (you can find this using
the ifconfig or ip addr command) and replace "00:11:22:33:44:55" with the MAC address you want to set.
Step 4: Save the script with a .py extension, for example, change_mac.py.
Step 5: Open a terminal and run the script using python change_mac.py.
This code will temporarily change the MAC address of the specified network interface. Please use it responsibly and for lawful purposes.
Method 2.
import string
import random
class MacAddressGenerator:
def __init__(self):
self.uppercased_hexdigits = ''.join(set(string.hexdigits.upper())
def generate_random_mac_address(self):
mac = ""
for i in range(6):
for j in range(2):
if i == 0:
mac += random.choice("02468ACE")
else:
mac += random.choice(self.uppercased_hexdigits)
mac += ":"
return mac.strip(":")
# Example usage:
mac_generator = MacAddressGenerator()
random_mac = mac_generator.generate_random_mac_address()
print(random_mac)
We define a class named MacAddressGenerator to encapsulate the MAC address generation logic.
In the class constructor, __init__, we initialize an instance variable uppercased_hexdigits. This variable contains all the uppercase hexadecimal digits (0-9 and A-F) and is used to ensure that we generate valid MAC address characters.
The generate_random_mac_address method generates a random MAC address. Here's a step-by-step explanation of what this method does: a. Initialize an empty string mac to store the MAC address. b. Iterate six times (for the six pairs of characters in a MAC address). c. For each pair, iterate twice (for the two characters in the pair). d. For the first character of the pair (at i == 0), select a random character from the set "02468ACE." This is because the second character of a MAC address must be one of these values. e. For the second character of the pair, select a random uppercase hexadecimal digit from uppercased_hexdigits. f. Add a colon ":" between each pair of characters. g. Finally, strip the trailing colon from the MAC address string.
After defining the MacAddressGenerator class, we create an instance of it (mac_generator) and use the generate_random_mac_address method to obtain a random MAC address.
The generated MAC address is printed to the console.
Comments