Build A Nmap For Open Ports
- Swift Glitxh
- Oct 14, 2023
- 2 min read
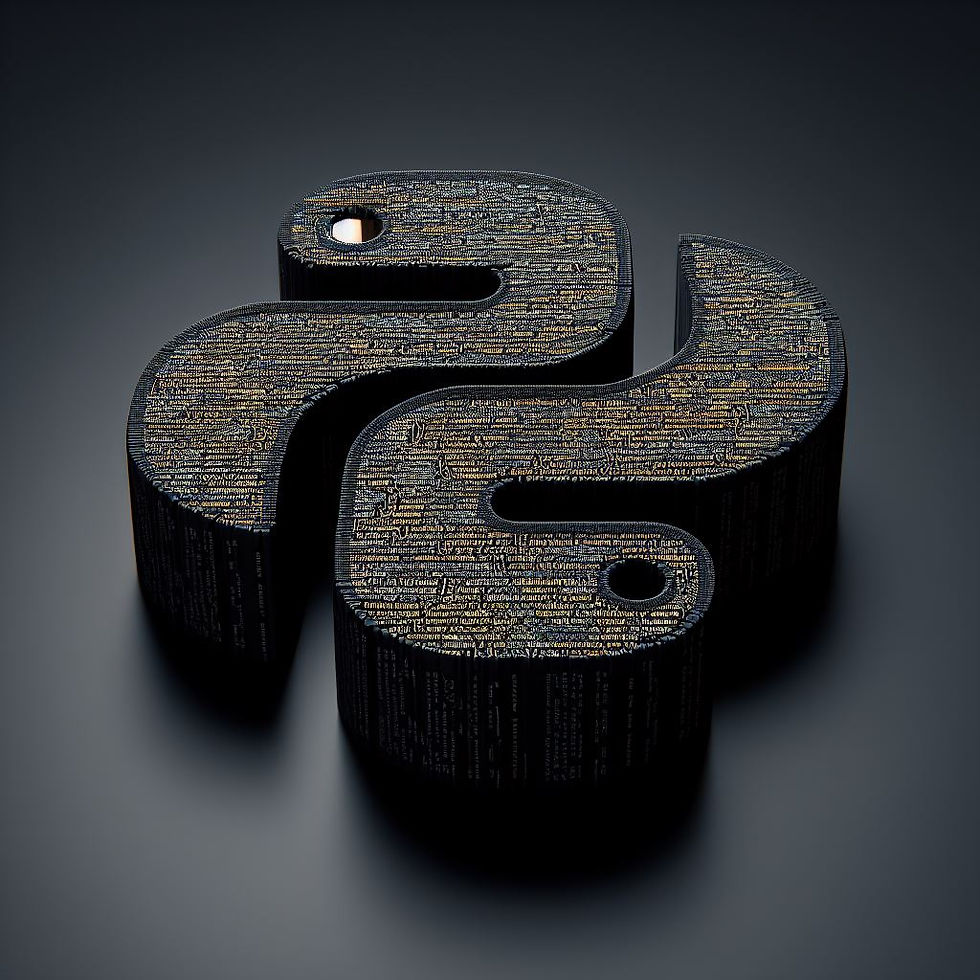
Step 1: Writing the Scanner
Let's start by creating a basic Python script for a simple port scanner.
Step 2: Running the Scanner
Replace "example.com" with the website/IP address you want to scan, and adjust the
ports_to_scan range as needed. Save the script to a Python file (e.g., port_scanner.py)
and run it.
import nmap # Define the target IP address or hostname target_ip = "example.com" # Create an Nmap PortScanner object nm = nmap.PortScanner() # Perform a simple Nmap scan on the target IP nm.scan(hosts=target_ip, arguments='-F') # Print the scan results for host in nm.all_hosts(): print(f"Scanning host: {host}") for proto in nm[host].all_protocols(): print(f"Protocol: {proto}") port_list = list(nm[host][proto].keys()) port_list.sort() for port in port_list: state = nm[host][proto][port]['state'] print(f"Port {port}: {state}")
python port_scanner.py
Result
┌──(kali㉿kali)-[~]
└─$ python port_scanner.py
Scanning host: 93.184.216.34
Protocol: tcp
Port 7: open
Port 9: open
Port 13: open
Port 21: open
Port 22: open
Port 23: open
Port 25: open
Port 26: open
Port 37: open
Port 53: open
Port 79: open
Port 80: open
Port 81: open
Port 88: open
...
The Breakdown
Import the nmap Library: First, you need to import the nmap library into your Python script. This library provides a Pythonic interface to Nmap, a popular network scanning tool.
Define the Target IP Address: You specify the target IP address or hostname that you want to scan. This can be the IP address of a remote server, a website, or any reachable network device. You replace the placeholder with the actual target IP or hostname.
Create a PortScanner Object: You create an instance of the PortScanner class from the nmap library. This object will be used to perform the scan on the target IP address.
Perform the Nmap Scan: You initiate the Nmap scan by calling the scan method of the PortScanner object. This method takes two main arguments: hosts: The target IP address or hostname to scan. arguments: The scan options and flags to specify how the scan should be conducted. In the provided example, -F is used to perform a fast scan on the 100 most common ports. You can customize the scan options according to your needs
Print Scan Results: After the scan is complete, you can access the results using the PortScanner object. You iterate through the scanned hosts and protocols to access information about open ports. The script prints details such as the host being scanned, the detected protocol (e.g., TCP or UDP), and the state of open ports (e.g., "open" or "closed").
留言