Write A Directory Brute-Force Tool In Python
- Swift Glitxh
- Oct 13, 2023
- 2 min read
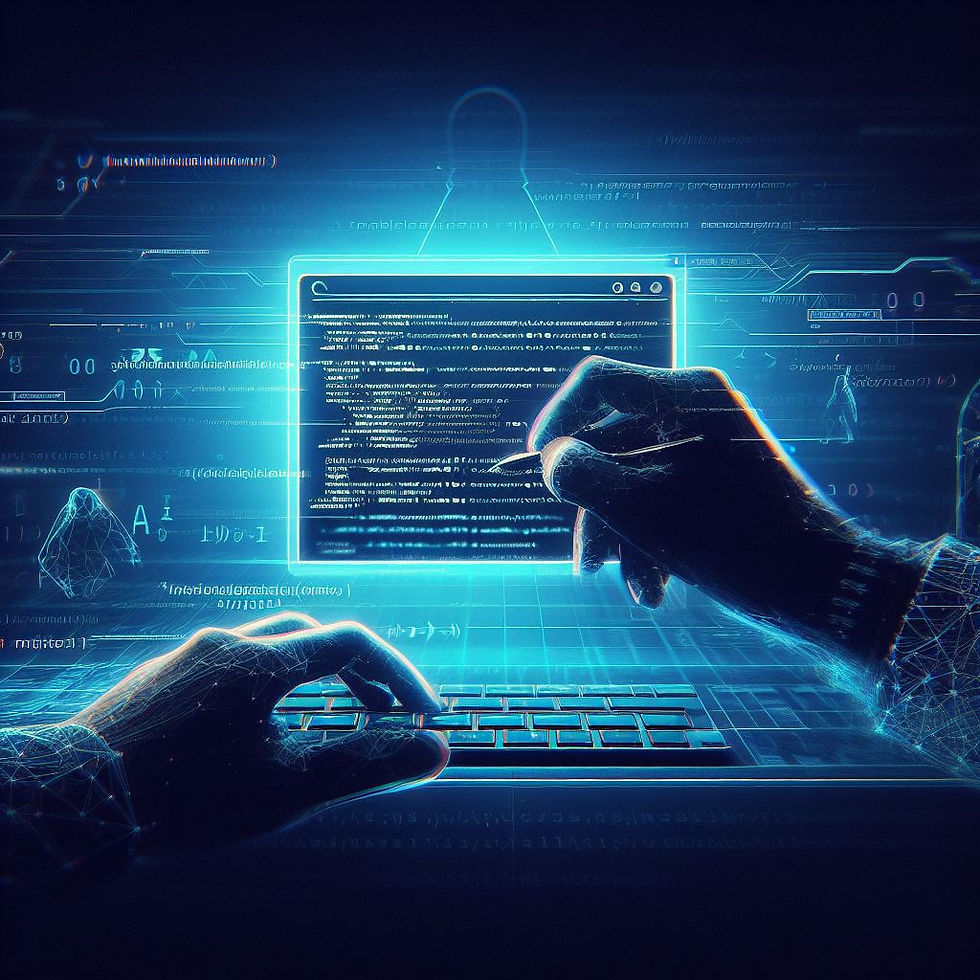
Step 1: Prepare Your Environment
Make sure you have Python installed on your system. You'll also need to install the requests library if you haven't already. You can install it using pip:
pip install requests
Step 2: Create a File with Directory Names
Create a text file named "directories.txt" and list the directory names you want to brute-force, with one directory name per line. For example:
directories.txt
admin
uploads
images
config
Step 3: Modify the Script
Copy the following Python script and modify the target_url variable with the URL you want to perform the directory brute-force on. Ensure that the directory_file variable matches the name of the file you created in Step 2.
python
import requests
# Target URL to perform directory brute-force on
target_url = "https://example.com/"# Function to read directory names from a filedef read_directories_from_file(filename):
with open(filename, "r") as file:
directories = [line.strip() for line in file.readlines()]
return directories
# Function to perform directory brute-forcedef dir_bruteforce(url, directories):
for directory in directories:
full_url = url + directory
response = requests.get(full_url)
if response.status_code == 200:
print(f"[+] Found: {full_url}")
elif response.status_code == 403:
print(f"[!] Access denied: {full_url}")
else:
print(f"[-] Not found: {full_url}")
# File containing directory names
directory_file = "directories.txt"# Read directory names from the file
common_directories = read_directories_from_file(directory_file)
# Perform directory brute-force
dir_bruteforce(target_url, common_directories)
Step 4: Run the Script
Open your terminal or command prompt, navigate to the directory where you saved the script and the "directories.txt" file, and run the script:
python script_name.py
Replace "script_name.py" with the actual name of your Python script.
Step 5: Review the Results
The script will attempt to access each URL formed by combining the target URL and the directories listed in the file. It will print whether each directory was found (status code 200), if access was denied (status code 403), or if the directory was not found (status code 404).
Please ensure that you have proper authorization to perform directory brute-forcing on the target URL, as unauthorized scanning of websites may violate legal and ethical standards. Always use such tools responsibly and with permission.
Comments